#include "pass_bits/helper/astro_problems/astro_functions.hpp"
#include "pass_bits/helper/astro_problems/lambert.hpp"
#include "pass_bits/helper/astro_problems/mga_dsm.hpp"
#include "pass_bits/helper/astro_problems/pl_eph_an.hpp"
#include "pass_bits/helper/astro_problems/propagate_kep.hpp"
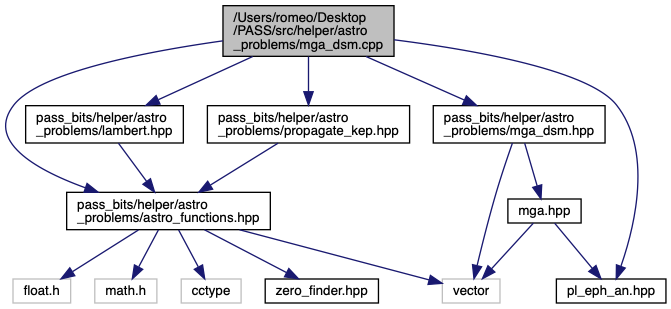
Functions | |
void | vector_normalize (const double in[3], double out[3]) |
void | get_celobj_r_and_v (const mgadsmproblem &problem, const double T, const int i_count, double *r, double *v) |
void | precalculate_ers_and_vees (const std::vector< double > &t, const mgadsmproblem &problem, std::vector< double *> &r, std::vector< double *> &v) |
double | get_celobj_mu (const mgadsmproblem &problem, const int i_count) |
void | first_block (const std::vector< double > &t, const mgadsmproblem &problem, const std::vector< double *> &r, std::vector< double *> &v, std::vector< double > &DV, double v_sc_nextpl_in[3]) |
void | intermediate_block (const std::vector< double > &t, const mgadsmproblem &problem, const std::vector< double *> &r, const std::vector< double *> &v, int i_count, const double v_sc_pl_in[], std::vector< double > &DV, double *v_sc_nextpl_in) |
void | final_block (const mgadsmproblem &problem, const std::vector< double *> &v, const double v_sc_pl_in[], std::vector< double > &DV) |
double | time2distance (const double *r0, const double *v0, double rtarget) |
int | MGA_DSM (std::vector< double > t, mgadsmproblem &problem, double &J) |
Variables | |
const double | MU [9] |
const double | RPL [6] |
Function Documentation
◆ final_block()
void final_block | ( | const mgadsmproblem & | problem, |
const std::vector< double *> & | v, | ||
const double | v_sc_pl_in[], | ||
std::vector< double > & | DV | ||
) |

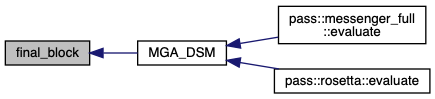
◆ first_block()
void first_block | ( | const std::vector< double > & | t, |
const mgadsmproblem & | problem, | ||
const std::vector< double *> & | r, | ||
std::vector< double *> & | v, | ||
std::vector< double > & | DV, | ||
double | v_sc_nextpl_in[3] | ||
) |
t - decision vector problem - problem parameters r - planet positions v - planet velocities DV - [output] velocity contributions table v_sc_pl_in - [output] next hop input speed
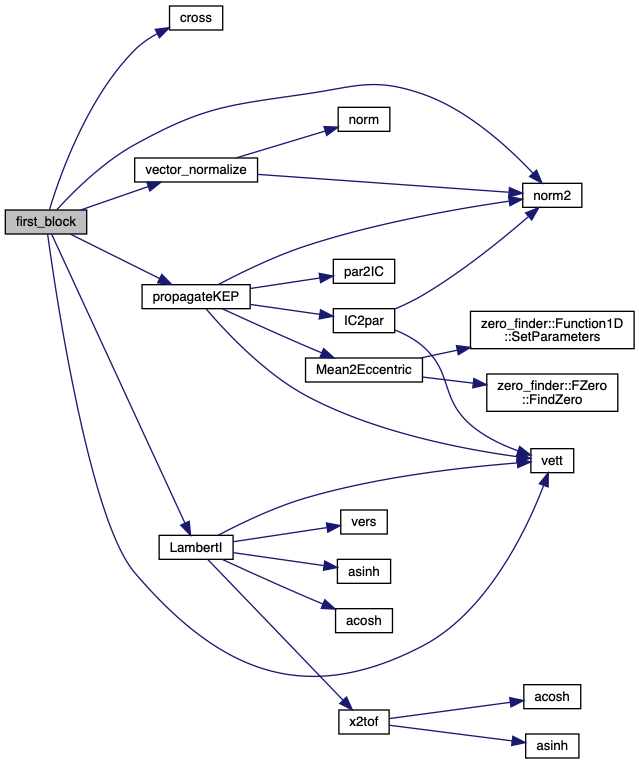
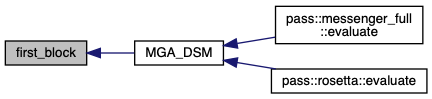
◆ get_celobj_mu()
double get_celobj_mu | ( | const mgadsmproblem & | problem, |
const int | i_count | ||
) |
Get gravitational constant of an celestial object of interest.
problem - concerned problem i_count - hop number (starting from 0)

◆ get_celobj_r_and_v()
void get_celobj_r_and_v | ( | const mgadsmproblem & | problem, |
const double | T, | ||
const int | i_count, | ||
double * | r, | ||
double * | v | ||
) |
Compute velocity and position of an celestial object of interest at specified time.
problem - concerned problem T - time i_count - hop number (starting from 0) r - [output] object's position v - [output] object's velocity


◆ intermediate_block()
void intermediate_block | ( | const std::vector< double > & | t, |
const mgadsmproblem & | problem, | ||
const std::vector< double *> & | r, | ||
const std::vector< double *> & | v, | ||
int | i_count, | ||
const double | v_sc_pl_in[], | ||
std::vector< double > & | DV, | ||
double * | v_sc_nextpl_in | ||
) |
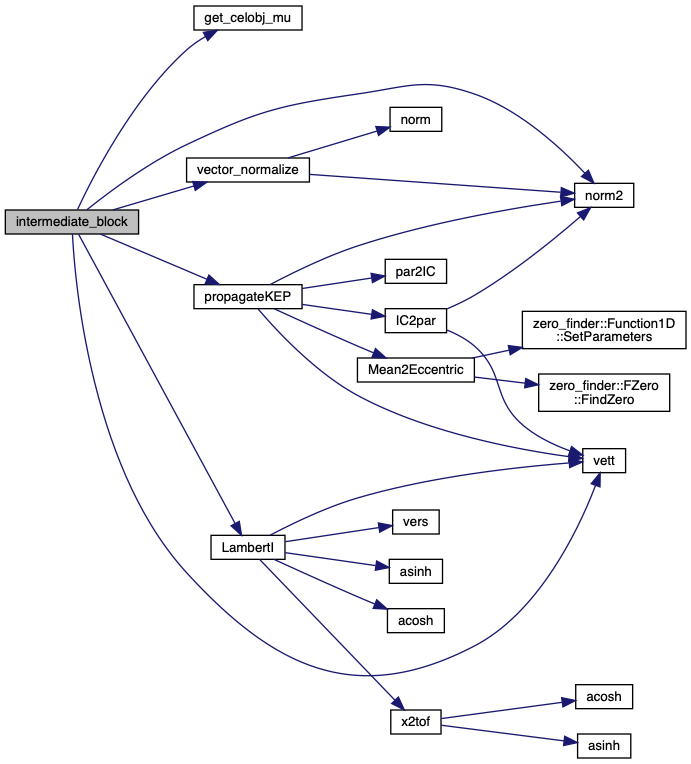
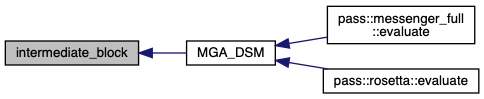
◆ MGA_DSM()
int MGA_DSM | ( | std::vector< double > | t, |
mgadsmproblem & | problem, | ||
double & | J | ||
) |
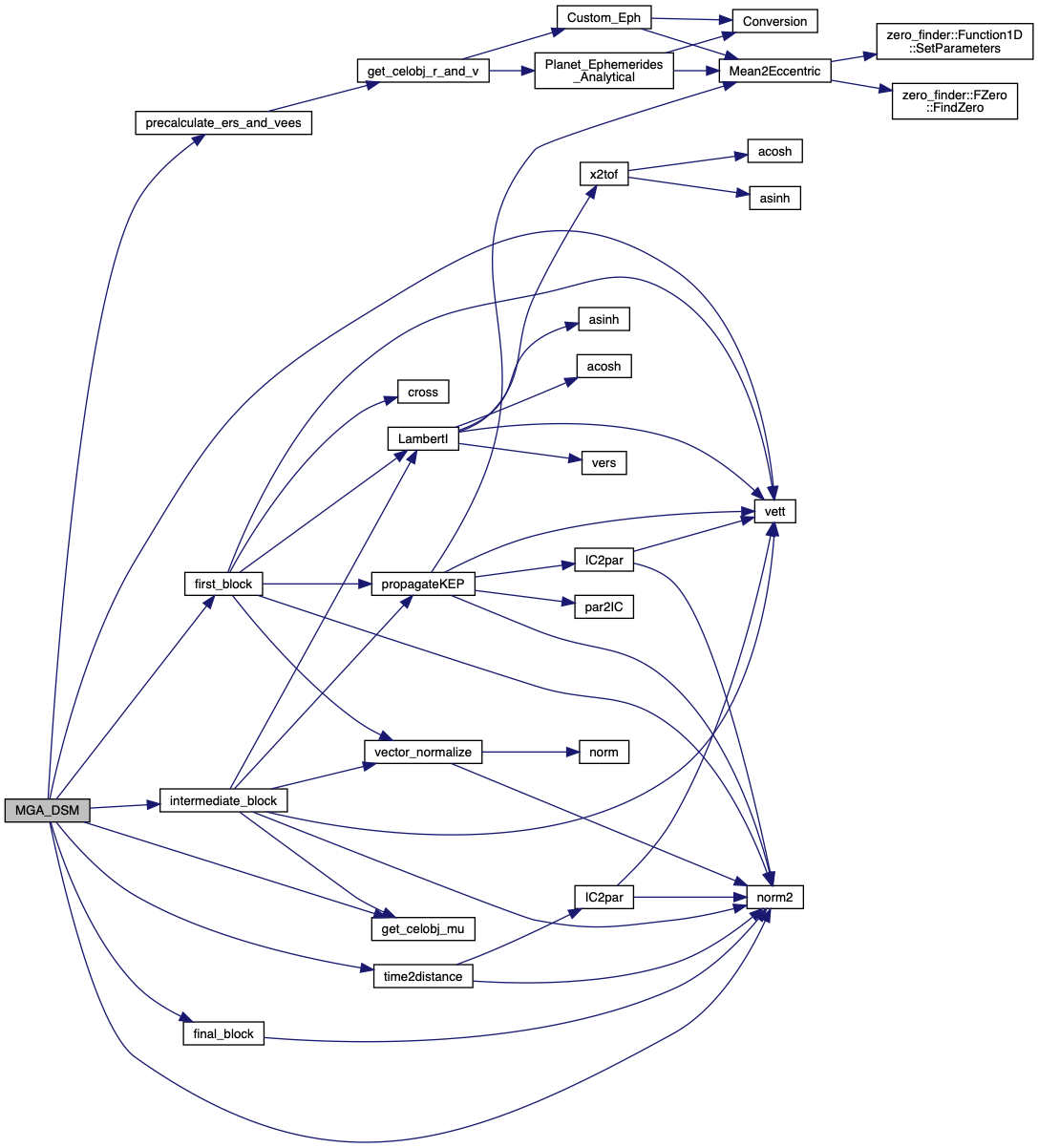
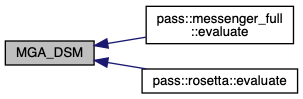
◆ precalculate_ers_and_vees()
void precalculate_ers_and_vees | ( | const std::vector< double > & | t, |
const mgadsmproblem & | problem, | ||
std::vector< double *> & | r, | ||
std::vector< double *> & | v | ||
) |
Precomputes all velocities and positions of celestial objects of interest for the problem. Before calling this function, r and v verctors must be pre-allocated with sufficient amount of entries.
problem - concerned problem r - [output] array of position vectors v - [output] array of velocity vectors


◆ time2distance()
double time2distance | ( | const double * | r0, |
const double * | v0, | ||
double | rtarget | ||
) |
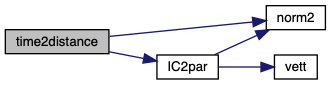
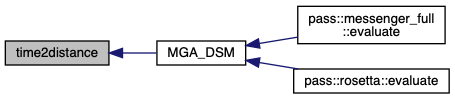
◆ vector_normalize()
void vector_normalize | ( | const double | in[3], |
double | out[3] | ||
) |
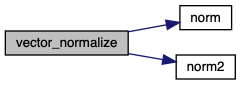

Variable Documentation
◆ MU
const double MU[9] |
◆ RPL
const double RPL[6] |