#include <problem.hpp>
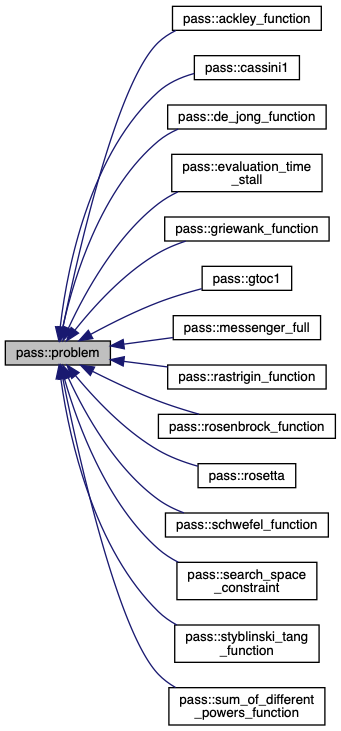
Public Member Functions | |
arma::vec | bounds_range () const noexcept |
arma::uword | dimension () const noexcept |
problem (const arma::uword dimension, const double lower_bound, const double upper_bound, const std::string &name) | |
problem (const arma::vec &lower_bounds, const arma::vec &upper_bounds, const std::string &name) | |
virtual double | evaluate (const arma::vec &agent) const =0 |
double | evaluate_normalised (const arma::vec &normalised_agent) const |
arma::mat | normalised_random_agents (const arma::uword count) const |
arma::mat | normalised_hammersley_agents (const arma::uword count) const |
arma::mat | initialise_normalised_agents (const arma::uword count) const |
Public Attributes | |
const arma::vec | lower_bounds |
const arma::vec | upper_bounds |
const std::string | name |
Detailed Description
This class defines the interface for problems that can be approximated by a pass::optimiser
. Subclasses need only implement evaluate
.
Constructor & Destructor Documentation
◆ problem() [1/2]
pass::problem::problem | ( | const arma::uword | dimension, |
const double | lower_bound, | ||
const double | upper_bound, | ||
const std::string & | name | ||
) |
Initialises an dimension
-dimensional problem with uniform lower and upper bounds and a problem name.
◆ problem() [2/2]
pass::problem::problem | ( | const arma::vec & | lower_bounds, |
const arma::vec & | upper_bounds, | ||
const std::string & | name | ||
) |
Initialises this problem with custom lower and upper bounds and a problem name. The problem dimension is derived from the bounds dimensions, which have to be equal.

Member Function Documentation
◆ bounds_range()
|
noexcept |
Returns the element-wise maximum difference between upper_bounds
and lower_bounds
.

◆ dimension()
|
noexcept |
Returns the problem dimension.
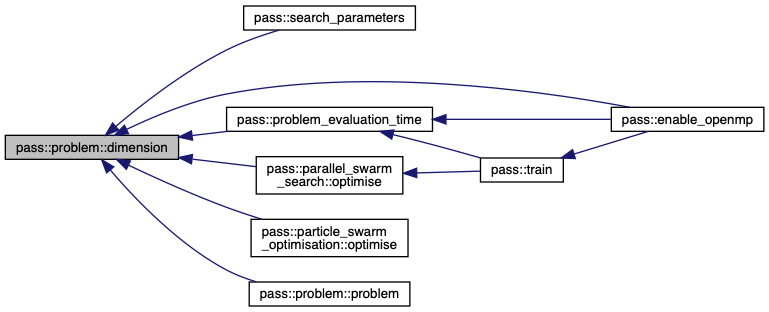
◆ evaluate()
|
pure virtual |
Evaluates this problem at agent
, which must match the dimensions of this problem.
Implemented in pass::gtoc1, pass::rosenbrock_function, pass::rastrigin_function, pass::schwefel_function, pass::ackley_function, pass::styblinski_tang_function, pass::search_space_constraint, pass::de_jong_function, pass::griewank_function, pass::sum_of_different_powers_function, pass::evaluation_time_stall, pass::cassini1, pass::messenger_full, and pass::rosetta.
◆ evaluate_normalised()
double pass::problem::evaluate_normalised | ( | const arma::vec & | normalised_agent | ) | const |
Evaluates this problem at agent
, which must be a normalized vector (all values must be in range [0, 1]). agent
is mapped to the problem boundaries before evaluation.
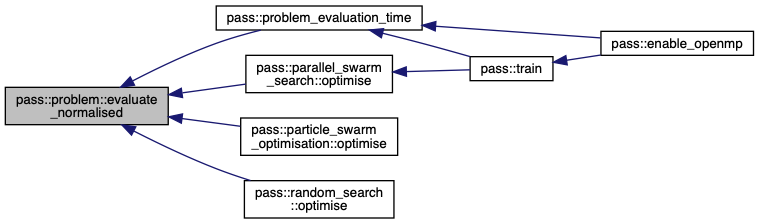
◆ initialise_normalised_agents()
arma::mat pass::problem::initialise_normalised_agents | ( | const arma::uword | count | ) | const |
Draws count
distributed random agents from range [0, 1], stored column-wise. Agens are from type hammersley or random distributed (50%-50%)

◆ normalised_hammersley_agents()
arma::mat pass::problem::normalised_hammersley_agents | ( | const arma::uword | count | ) | const |
Generates count
hammersley points with the same dimension as this, stored column-wise.
For a definition of hammersley points, see http://www.cse.cuhk.edu.hk/~ttwong/papers/udpoint/udpoint.pdf, equations 1 to 3.

◆ normalised_random_agents()
arma::mat pass::problem::normalised_random_agents | ( | const arma::uword | count | ) | const |
Draws count
uniformly distributed random agents from range [0, 1], stored column-wise.
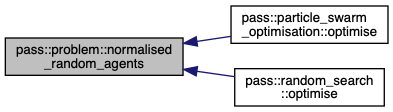
Member Data Documentation
◆ lower_bounds
const arma::vec pass::problem::lower_bounds |
Lower bound constraints for each problem dimension. An optimiser will never evaluate a point outside of these bounds.
Must be element-wise less than upper_bounds
.
◆ name
const std::string pass::problem::name |
Identify every problem with its own name
◆ upper_bounds
const arma::vec pass::problem::upper_bounds |
Upper bound constraints for each problem dimension. An optimiser will never evaluate a point outside of these bounds.
Must be element-wise greater than upper_bounds
.
The documentation for this class was generated from the following files:
- /Users/romeo/Desktop/PASS/include/pass_bits/problem.hpp
- /Users/romeo/Desktop/PASS/src/problem.cpp