Namespaces | |
gtoc | |
Classes | |
class | ackley_function |
struct | asteroid |
class | cassini1 |
struct | celestial_body |
class | de_jong_function |
class | evaluation_time_stall |
class | griewank_function |
class | gtoc1 |
struct | lambert_solution |
class | messenger_full |
struct | optimise_result |
class | optimiser |
class | parallel_swarm_search |
class | particle_swarm_optimisation |
class | problem |
class | random_search |
class | rastrigin_function |
class | regression |
class | rosenbrock_function |
class | rosetta |
class | schwefel_function |
class | search_space_constraint |
class | seed |
class | stopwatch |
class | styblinski_tang_function |
class | sum_of_different_powers_function |
Typedefs | |
typedef struct pass::lambert_solution | lambert_solution |
Functions | |
void | search_parameters (const pass::problem &problem, const bool benchmark) |
arma::mat | parameter_evaluate (pass::optimiser &optimiser, const pass::problem &problems) |
arma::uword | compare_segments (const arma::mat first_segment_runtimes, const arma::mat second_segment_runtimes) |
bool | enable_openmp (const pass::problem &problem) |
arma::mat | train (const int &examples) |
arma::rowvec | build_model (const arma::mat &training_points) |
double | problem_evaluation_time (const pass::problem &problem) |
int | thread_number () |
int | number_of_threads () |
int | node_rank () |
int | number_of_nodes () |
lambert_solution | lambert (std::array< double, 3 > r1_in, std::array< double, 3 > r2_in, double t, const double mu, const int lw) |
std::pair< double, double > | pow_swing_by_inv (const double Vin, const double Vout, const double alpha) |
std::pair< std::array< double, 3 >, std::array< double, 3 > > | conversion (const std::array< double, 6 > &E, const double mu) |
double | mean_to_eccentric (const double m, const double e) |
void | pow_swing_by_inv (const double, const double, const double, double &, double &) |
double | random_double_uniform_in_range (double min, double max) |
int | random_integer_uniform_in_range (int min, int max) |
arma::rowvec | integers_uniform_in_range (const int min, const int max, const int count) |
arma::vec | random_neighbour (const arma::vec &agent, const double minimal_distance, const double maximal_distance) |
bool | is_verbose (false) |
int | global_number_of_runs (1) |
double | precision (1e-06) |
arma::uword | parameter_setting_number_of_runs (10) |
Variables | |
bool | is_verbose |
int | global_number_of_runs |
double | precision |
arma::uword | parameter_setting_number_of_runs |
const std::array< arma::uword, 2000 > | prime_numbers |
Typedef Documentation
◆ lambert_solution
typedef struct pass::lambert_solution pass::lambert_solution |
Function Documentation
◆ build_model()
arma::rowvec pass::build_model | ( | const arma::mat & | training_points | ) |
Machine Learning - Step 2.
Builds the model from the trainingdata. Tests two models: linear and polynomial.
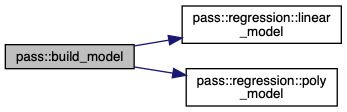

◆ compare_segments()
arma::uword pass::compare_segments | ( | const arma::mat | first_segment_runtimes, |
const arma::mat | second_segment_runtimes | ||
) |
Compare the two segments with each other.
- Returns
- '1' if the first segmet is the best
- '2' if the second segmet is the best

◆ conversion()
std::pair< std::array< double, 3 >, std::array< double, 3 > > pass::conversion | ( | const std::array< double, 6 > & | E, |
const double | mu | ||
) |
This function is an adapted version of function Conversion
in file ./astro_functions.hpp
.
Returns a tuple (position, velocity).
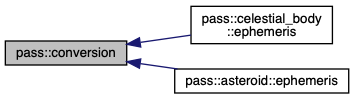
◆ enable_openmp()
bool pass::enable_openmp | ( | const pass::problem & | problem | ) |
Depending on your problem it returns if you should use openMP or not Outputs are given through all the analyse process.
Steps:
- Estimate the evaluation time of your problem
- Generate trainingsdata
- Test linear model if it fits
- Test polynomial model if it fitst
- Predict the speedup for your model
- Give suggestions if to activate openMP or not
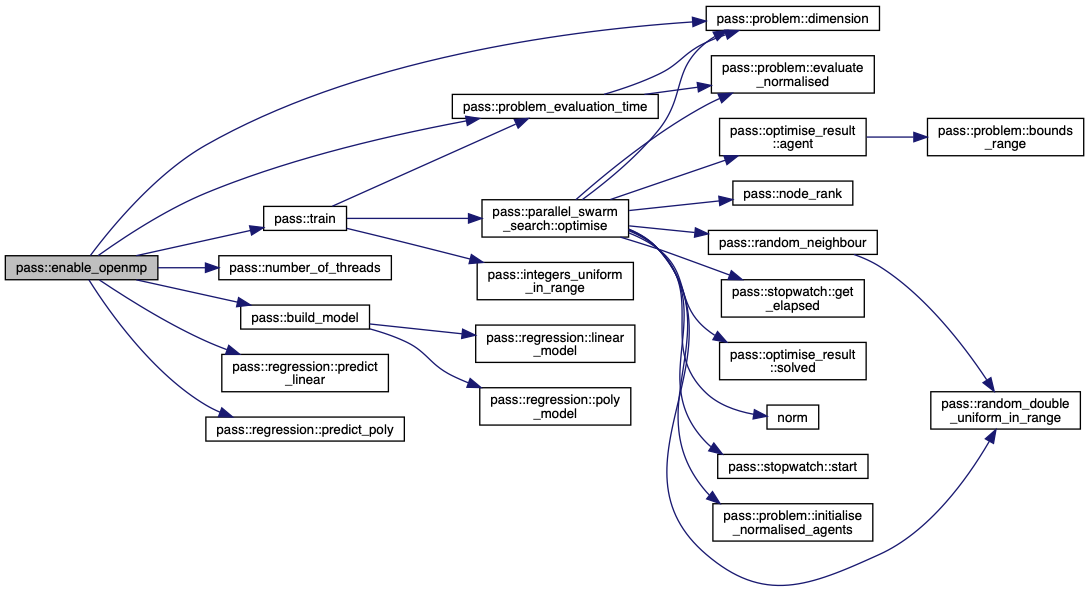
◆ global_number_of_runs()
int pass::global_number_of_runs | ( | 1 | ) |
Global variables used for evaluations : to help saving raw data Starts from '1'
◆ integers_uniform_in_range()
arma::rowvec pass::integers_uniform_in_range | ( | const int | min, |
const int | max, | ||
const int | count | ||
) |
Returns a uniformly 'count' drawn random integer numbers in range [min, max].

◆ is_verbose()
bool pass::is_verbose | ( | false | ) |
Global variables used for evaluations : analyse the behaviour of the algorithm Is initialized to false
.
◆ lambert()
pass::lambert_solution pass::lambert | ( | std::array< double, 3 > | r1_in, |
std::array< double, 3 > | r2_in, | ||
double | t, | ||
const double | mu, | ||
const int | lw | ||
) |
This function is an adapted version of function LambertI
in file ./lambert.h
. For more details and documentation, please take a look at the original file.
Throws std::invalid_argument
if t
is negative or zero.
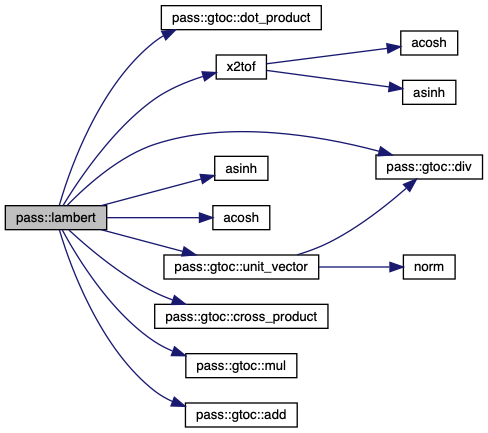

◆ mean_to_eccentric()
double pass::mean_to_eccentric | ( | const double | m, |
const double | e | ||
) |
This function is an adapted version of function Mean2Eccentric
in file ./astro_functions.h
.
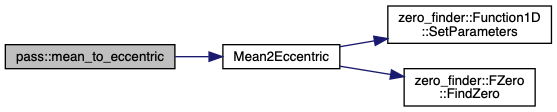
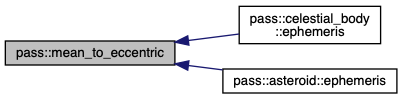
◆ node_rank()
int pass::node_rank | ( | ) |
Use MPI : returns which number a.k.a rank the node have

◆ number_of_nodes()
int pass::number_of_nodes | ( | ) |
Use MPI : returns the number of the nodes

◆ number_of_threads()
int pass::number_of_threads | ( | ) |
Use OpenMP : returns the number of the threads

◆ parameter_evaluate()
arma::mat pass::parameter_evaluate | ( | pass::optimiser & | optimiser, |
const pass::problem & | problems | ||
) |
Evaluate the Problem.
The Problem will be evaluated 10 times. Set the global variable to change that pass::parameter_setting_number_of_runs
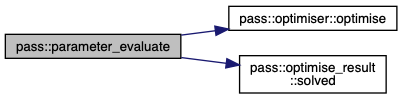

◆ parameter_setting_number_of_runs()
arma::uword pass::parameter_setting_number_of_runs | ( | 10 | ) |
Global variables used for evaluations : number of the runs Is initialized to 10
.
◆ pow_swing_by_inv() [1/2]
void pass::pow_swing_by_inv | ( | const double | Vin, |
const double | Vout, | ||
const double | alpha, | ||
double & | DV, | ||
double & | rp | ||
) |
◆ pow_swing_by_inv() [2/2]
std::pair< double, double > pass::pow_swing_by_inv | ( | const double | Vin, |
const double | Vout, | ||
const double | alpha | ||
) |
This function is an adapted version of function pow_swing_by_inv
in file ./pow_swing_by_inv.hpp
.
Returns a tuple (DV, rp).

◆ precision()
double pass::precision | ( | 1e- | 06 | ) |
Global variables used for evaluations : the precision to stop algorithms and set restart Is initialized to 1e-06
.
◆ problem_evaluation_time()
double pass::problem_evaluation_time | ( | const pass::problem & | problem | ) |
Returns the evaluation time of a problem in nanoseconds.
NOTE: It is not a requirement to get the same output always because the CPU can be less or more used by other processes running on the computer. In the human mind, we can remember the solution of a math problem, though for a computer the same process will always be something new, so, it is not required to get the same result always!
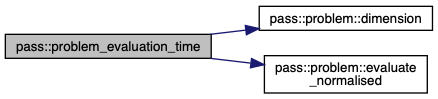

◆ random_double_uniform_in_range()
double pass::random_double_uniform_in_range | ( | double | min, |
double | max | ||
) |
Returns a uniformly drawn random double number in range [min, max].

◆ random_integer_uniform_in_range()
int pass::random_integer_uniform_in_range | ( | int | min, |
int | max | ||
) |
Returns a uniformly drawn random integer number in range [min, max].
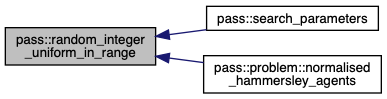
◆ random_neighbour()
arma::vec pass::random_neighbour | ( | const arma::vec & | agent, |
const double | minimal_distance, | ||
const double | maximal_distance | ||
) |
Return a randomly and uniformly drawn neighbour of agent, with distance [minimal_distance, maximal_distance].


◆ search_parameters()
void pass::search_parameters | ( | const pass::problem & | problem, |
const bool | benchmark | ||
) |
Search the best parameters for the parallel swarm search algorithm and saves the parameters in a file which can be than loaded.
Following parameter of the parallel swarm search are investigated:
- Swarm Size
- Neighbourhood Probability
- Inertia
- Cognitive Acceleration = Social Acceleration
- problem: the given problem
- Benchmark? 'True' or 'False'
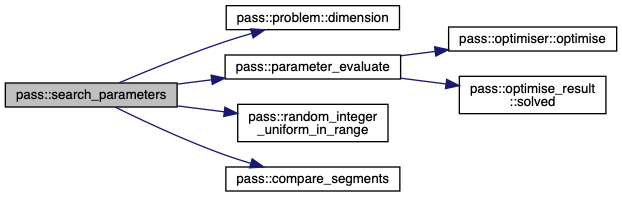
◆ thread_number()
int pass::thread_number | ( | ) |
Use OpenMP : returns which number the thread have
◆ train()
arma::mat pass::train | ( | const int & | examples | ) |
Machine Learning - Step 1.
Collects trainingdata for the model. Default: 120 trainingspoint are collected The more data you collect (better data), the better the model performs.
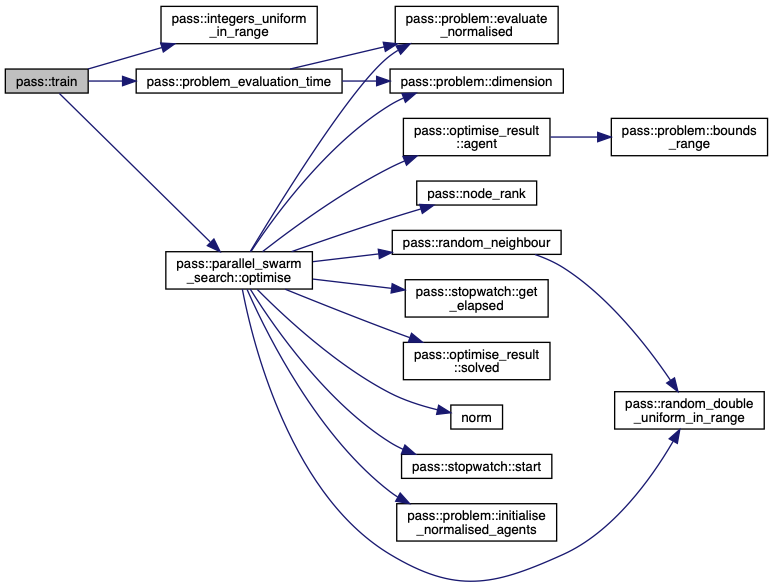

Variable Documentation
◆ global_number_of_runs
int pass::global_number_of_runs |
Global variables used for evaluations : to help saving raw data Starts from '1'
◆ is_verbose
bool pass::is_verbose |
Global variables used for evaluations : analyse the behaviour of the algorithm Is initialized to false
.
◆ parameter_setting_number_of_runs
arma::uword pass::parameter_setting_number_of_runs |
Global variables used for evaluations : number of the runs Is initialized to 10
.
◆ precision
double pass::precision |
Global variables used for evaluations : the precision to stop algorithms and set restart Is initialized to 1e-06
.
◆ prime_numbers
const std::array< arma::uword, 2000 > pass::prime_numbers |
Primes for use in pass::problem::hammersley_agents
.
Taken from https://cis.temple.edu/~beigel/cis573/alizzy/prime-list.html